Sequence 3 - Lesson 2: If-Then-Else
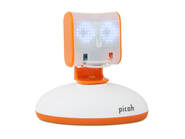
Learning Intention
I can use an if-then-else instruction in my program.
I can use an if-then-else instruction in my program.
Introduction
Conditionals are instructions in a program that perform different actions depending on whether their condition is true or false. They take in information and then depending on its content make a decision to do or not do something. You may be able to think of conditionals that you have encountered in real life. For example. If it is a school day you get up early.
This has a condition and then an action:
If (it is a school day)
Then (you get up early)
Point out that this does not confirm what will happen if it isn’t a school day. In other words it doesn’t say that you don’t get up early. Only that if it is a school day you do!
We are going to program Picoh to run a maths quiz and respond in different ways depending on the answer given. Let’s start by programming Picoh to receive an input from the computer keyboard. Show the program below and ask students to predict what will happen when the Green Flag is clicked?
Conditionals are instructions in a program that perform different actions depending on whether their condition is true or false. They take in information and then depending on its content make a decision to do or not do something. You may be able to think of conditionals that you have encountered in real life. For example. If it is a school day you get up early.
This has a condition and then an action:
If (it is a school day)
Then (you get up early)
Point out that this does not confirm what will happen if it isn’t a school day. In other words it doesn’t say that you don’t get up early. Only that if it is a school day you do!
We are going to program Picoh to run a maths quiz and respond in different ways depending on the answer given. Let’s start by programming Picoh to receive an input from the computer keyboard. Show the program below and ask students to predict what will happen when the Green Flag is clicked?
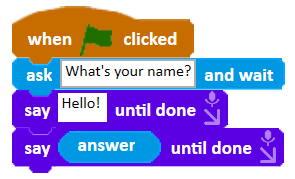
Ask students to try the code themselves. Can they explain how it works? Do they recognise the shape of the reporter block for answer? It is similar to the ones used in the previous lesson on time, but instead of reporting time or date, it reports the text that the user has typed in.
When the green flag is clicked the Ask and wait block will speak the words ‘What’s your name?’, then it will wait for text to be typed into the keyboard (and the enter key pressed). Then Picoh will say ‘Hello!’. And then because the ‘answer’ block has been dropped into the Say block it will speak the text that has been typed in. Each time the ask block runs and text is entered it will be returned as the typed in answer.
Highlight that it doesn’t have to be letters. If you type in an integer, it will also speak that. Let’s say my name was 11, for example… Remind children that this code simply enters data from the keyboard into the computer. A conditional is needed to decide what to do with the information that has been entered. We are going to use the If-then block. Show the diagram below that shows how this works.
When the green flag is clicked the Ask and wait block will speak the words ‘What’s your name?’, then it will wait for text to be typed into the keyboard (and the enter key pressed). Then Picoh will say ‘Hello!’. And then because the ‘answer’ block has been dropped into the Say block it will speak the text that has been typed in. Each time the ask block runs and text is entered it will be returned as the typed in answer.
Highlight that it doesn’t have to be letters. If you type in an integer, it will also speak that. Let’s say my name was 11, for example… Remind children that this code simply enters data from the keyboard into the computer. A conditional is needed to decide what to do with the information that has been entered. We are going to use the If-then block. Show the diagram below that shows how this works.
Teacher Input
Show this. Can they predict what will happen when the green flag is clicked and then the number 4 is entered? How about if the number 10 is entered, or the word cat? Ask children what the green operator block does in this program. Why is it needed?
Show this. Can they predict what will happen when the green flag is clicked and then the number 4 is entered? How about if the number 10 is entered, or the word cat? Ask children what the green operator block does in this program. Why is it needed?
Run the code and try entering different values. Did it do what you expected?
Activity 1
Ask children to modify the code to make Picoh ask a different question and give a different response.
Teacher Input 2
Currently any answer that is not 4 will get no response.
One way to give a response when a wrong answer is entered would be to have an if statement for every possible other answer.
Ask children to modify the code to make Picoh ask a different question and give a different response.
Teacher Input 2
Currently any answer that is not 4 will get no response.
One way to give a response when a wrong answer is entered would be to have an if statement for every possible other answer.
As you can see trying to cover even a few possible answers (including the rather unlikely ones) makes the program very long and it would be impossible to cover every answer this way.
Instead we can use an if-then-else conditional so that any answer other than the one that is true will result in running the instructions in the else part of the block.
Instead we can use an if-then-else conditional so that any answer other than the one that is true will result in running the instructions in the else part of the block.
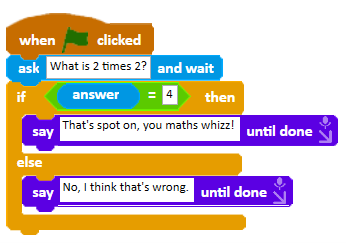
Show the example.
Activity 2
Can you modify the program created in Activity 1 to use an if-then-else conditional so that it gives a different response when the wrong answer is entered?
Activity 3
Can you write a program that makes Picoh ask a sequence of questions and give a response to each based on the answer entered? Here’s an example:
Can you modify the program created in Activity 1 to use an if-then-else conditional so that it gives a different response when the wrong answer is entered?
Activity 3
Can you write a program that makes Picoh ask a sequence of questions and give a response to each based on the answer entered? Here’s an example:
Note: Picoh can also be made to listen for answers using the Ask and Listen block. You will need to have Speech Recognition switched on. To do this click on the Search icon (magnifying glass). Type in Speech Privacy Settings and then move the toggle switch to on to activate speech recognition.
Extension 1:
What if we wanted to ask a question with a different type of answer? How about one that had a number of possible correct answers?
For example:
'Can you name one of Bart Simpson’s sisters?’
The answer could be either Lisa or Marge. We could use the OR operator block as the condition.
What if we wanted to ask a question with a different type of answer? How about one that had a number of possible correct answers?
For example:
'Can you name one of Bart Simpson’s sisters?’
The answer could be either Lisa or Marge. We could use the OR operator block as the condition.
The OR operator block gives a True response if the answer is EITHER Lisa or Marge.
Can you add a question that has several possible answers?
Can you add a question that has several possible answers?
Extension 2
How about a question with an answer that must include a number of different answers.
For example:
‘Can you name both of Bart Simpson’s sisters?’
The answer must include both Maggie and Lisa.
The Contains operator block can be used here. This gives a true if the answer has the word Maggie anywhere in it. When combined with the AND operator block as shown below it will give a True responses if the answer contains Maggie and Lisa.
How about a question with an answer that must include a number of different answers.
For example:
‘Can you name both of Bart Simpson’s sisters?’
The answer must include both Maggie and Lisa.
The Contains operator block can be used here. This gives a true if the answer has the word Maggie anywhere in it. When combined with the AND operator block as shown below it will give a True responses if the answer contains Maggie and Lisa.
Can you use the Contains and AND operator blocks to create a program where you check whether the answer includes both names?
Share the following program either on a screen or in the Picoh app. Challenge children to predict what it will do when different answers are entered. Run the program and enter different answers. Can they identify the bug and how to correct it?
The program uses AND rather than OR so will only give the correct response when all three country names are entered.
Solution below:
Share the following program either on a screen or in the Picoh app. Challenge children to predict what it will do when different answers are entered. Run the program and enter different answers. Can they identify the bug and how to correct it?
The program uses AND rather than OR so will only give the correct response when all three country names are entered.
Solution below: